There are several reasons you might want to get all WooCommerce orders for a customer in your shop. For example, you may want to find out if they’ve ever purchased a product, or do something as simple as count the number of orders they’ve placed with you.
Since orders are a custom post type, you can use get_posts to query orders, which is a pretty standard WP query that most developers are familiar with and can use. There are a couple things you should note though:
- Most of the time, people query
shop_order
as the post type. You want to usewc_get_order_types()
for the post type instead, as this will ensure that you have all order types available in the shop. Plugins can create their own order types, so this is a must for future-proofing your code. - The
post_status
is also something that’s easy to mess up. Order statuses (processing, completed, etc), are post statuses, so you can’t just used ‘publish’. You also don’t want to hard-code the core WooCommerce statuses, as plugins may have added additional ones. Usearray_keys( wc_get_order_statuses() )
for post statuses instead.
Launch your business in minutes with GoDaddy Airo™
If we want to query orders for a customer, we can check for the _customer_user
meta key (the customer id) in the order, and we’ll get orders that match our customer’s id.
Let’s say we want to get orders for a logged-in customer. The 'meta_key' => '_customer_user'
will be used as part of our query, and we’ll set 'meta_value' => get_current_user_id()
.
$customer_orders = get_posts( array(
'numberposts' => -1,
'meta_key' => '_customer_user',
'meta_value' => get_current_user_id(),
'post_type' => wc_get_order_types(),
'post_status' => array_keys( wc_get_order_statuses() ),
) );
Let’s go through a complete sample that will get the customer orders, count them, and display a notice to our loyal customers on the account page who’ve placed 5 or more orders.
Use Case: Welcome Message for Repeat Customers
Let’s get our current user:
$customer = wp_get_current_user();
And determine what number of orders indicates a “loyal” customer:
$loyal_count = 5;
We’ll then use our query above to get all of the WooCommerce orders for our customer.
As a final step, let’s build a welcome notice for the customer to show on the account page, and only show it if they’ve placed our minimum number of orders:
// Text for our "thanks for loyalty" message
$notice_text = sprintf( 'Hey %1$s 😀 We noticed you\'ve placed more than %2$s orders with us – thanks for being a loyal customer!', $customer->display_name, $loyal_count );
// Display our notice if the customer has at least 5 orders
if ( count( $customer_orders ) >= $loyal_count ) {
wc_print_notice( $notice_text, 'notice' );
}
Now to complete it, let’s put all of this together and add it to the My Account page:
function wc_get_customer_orders() {
// Get all customer orders
$customer_orders = get_posts( array(
'numberposts' => -1,
'meta_key' => '_customer_user',
'meta_value' => get_current_user_id(),
'post_type' => wc_get_order_types(),
'post_status' => array_keys( wc_get_order_statuses() ),
) );
$customer = wp_get_current_user();
// Order count for a "loyal" customer
$loyal_count = 5;
// Text for our "thanks for loyalty" message
$notice_text = sprintf( 'Hey %1$s 😀 We noticed you\'ve placed more than %2$s orders with us – thanks for being a loyal customer!', $customer->display_name, $loyal_count );
// Display our notice if the customer has at least 5 orders
if ( count( $customer_orders ) >= $loyal_count ) {
wc_print_notice( $notice_text, 'notice' );
}
}
add_action( 'woocommerce_before_my_account', 'wc_get_customer_orders' );
If the customer has placed more than 5 orders in the shop, a thank you message will be displayed:
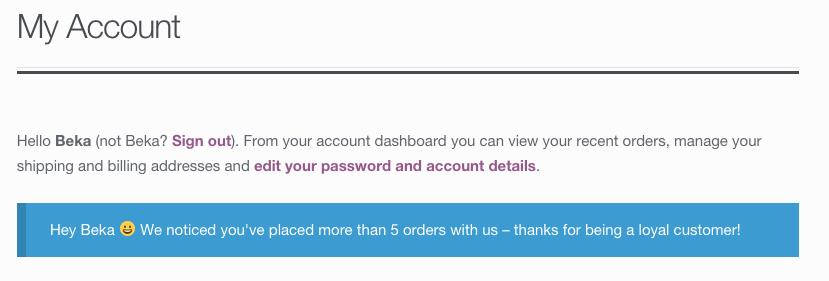
If not, they’ll see nothing.