When WooCommerce sends order emails to your customers, by default it only includes the product name in the order items table within the email.
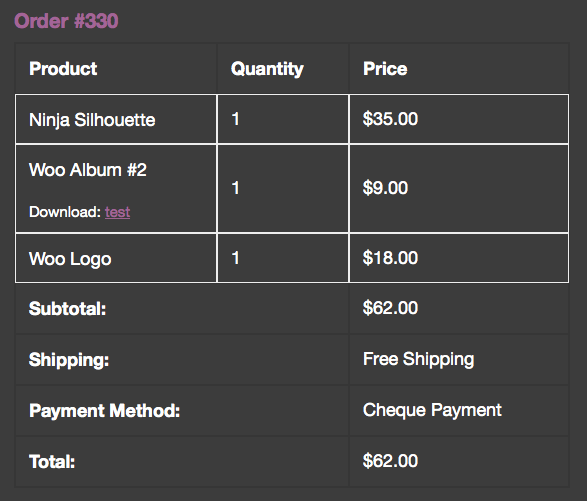
However, it’s possible to add some additional product details here, such as the product SKU or product featured image.
We’d previously written about adding information to WooCommerce order emails so that you could show product SKUs or product images within the WooCommerce order emails for customers. However, the process to do this was changed in a backwards-incompatible way in WooCommerce 2.5.
In previous versions of WooCommerce you were able to filter the entire email output, which made it possible to change whether images or SKUs were included.
However, with WooCommerce 2.5, the filter to change the order items table is still there, but it does not give you all of the data you need to make changes to the order items table in the email in the same way. You don’t have access to the $args
that are used in the template to determine what information to show or hide, so this filter pretty much doesn’t allow you to do anything directly.
If you’re already using WooCommerce 2.5, it’s possible to change the information shown in WooCommerce emails, we just have to be a bit more creative in how we use this filter. If you follow this tutorial, be sure you know how to add custom code to your website and that you’re using WooCommerce 2.5 or newer.
Add Product Images to WooCommerce Order Emails
You can add product images to to your order emails with this filter, but we can’t modify the arguments used to get the template directly. Instead, we’ll have to call the $order->email_order_items_table()
function again, but with new arguments to use. The reason this gets tricky is because if we call this function, it will call the filter we’re using, which will again call the function, which will again call our filter…you get the idea. We’ll get an infinite loop.
To modify the email output this way, we’ll need to use a static variable as a flag. This will let us only run our code once so that the email output is modified, then our code will bail out after it’s run the first time.
<?php // only copy this line if needed
/**
* Adds product images to the WooCommerce order emails table
* Uses WooCommerce 2.5 or newer
*
* @param string $output the buffered email order items content
* @param \WC_Order $order
* @return $output the updated output
*/
function sww_add_images_woocommerce_emails( $output, $order ) {
// set a flag so we don't recursively call this filter
static $run = 0;
// if we've already run this filter, bail out
if ( $run ) {
return $output;
}
$args = array(
'show_image' => true,
'image_size' => array( 100, 100 ),
);
// increment our flag so we don't run again
$run++;
// if first run, give WooComm our updated table
return $order->email_order_items_table( $args );
}
add_filter( 'woocommerce_email_order_items_table', 'sww_add_images_woocommerce_emails', 10, 2 );
That’s a wrap! This will change the way the email order items table is output to include product images when emails are sent to customers.
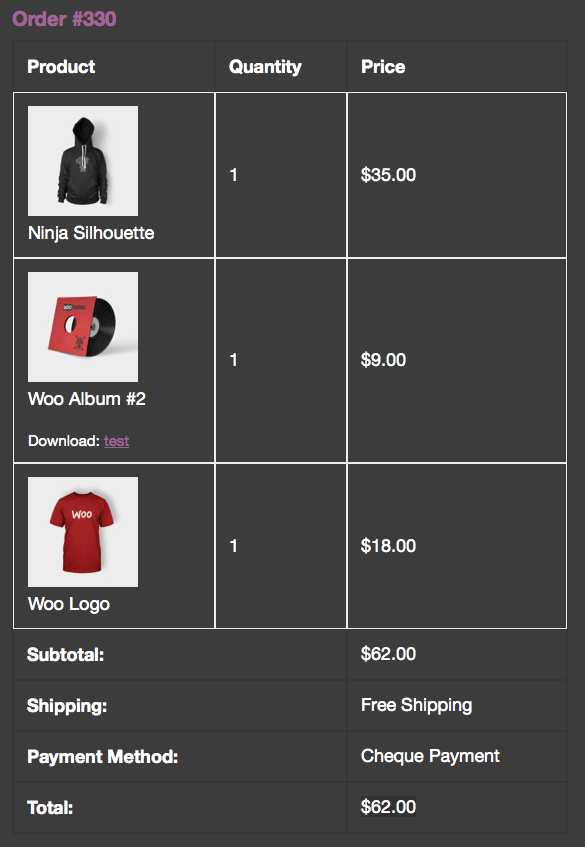
Add SKUs to WooCommerce Order Emails
We can use pretty much the exact same code to enable product SKUs in WooCommerce order emails instead of product images.
We’ll use the same snippet as above, but replace:
$args = array(
'show_image' => true,
'image_size' => array( 100, 100 ),
);
with:
$args = array(
'show_sku' => true,
);
This will add the SKU after the product name in this format: Product Name (#productSKU)
Here’s an example of what this would look like (the images wouldn’t be there of course):
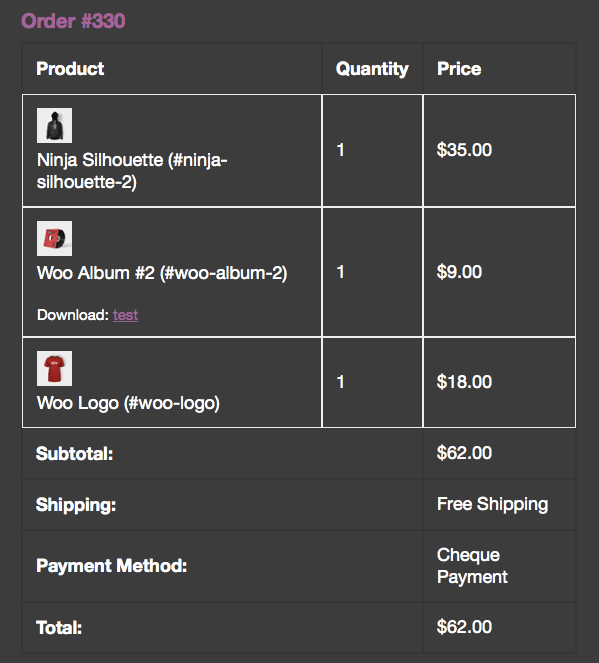
WooCommerce Order Emails: Show Both Images & SKUs
You can also add both SKUs and images to the order emails by adjusting the arguments to include both of them. The image size can be deleted if you want to use the default 32px by 32px size, or you can add your own size by adjusting the values below.
$args = array(
'show_sku' => true,
'show_image' => true,
'image_size' => array( 100, 100 ),
);
Add Product Information to WooCommerce Order Emails
Adding product SKUs or product images to WooCommerce order emails is possible, and you can add both if desired, along with changing the size of your image as needed. Remember, the code outlined above is only for WooCommerce 2.5 or newer.