If you’re wondering how to start a business in 2025, we’re here to help. In this guide, we’ll look at the essential steps you need to take to make your business a real success.
Many things go into starting and running a successful business. We’ll cover the initial planning of your new business, the legal aspects of starting a business, getting your finances in order and, finally, preparing for growth.
Launch your business in minutes with GoDaddy Airo™
Ready? Great. Let’s get started.
Disclaimer: This content should not be construed as legal or financial advice. Always consult an attorney or financial advisor regarding your specific legal or financial situation.
1. Find a business idea that will endure the ups and downs
First of all, when you're looking to create a business, you must find an idea that is feasible and something you feel passionate about.
Every budding business owner experiences a fear of failure. So landing on a business idea that you'll stick to no matter what is essential. To push through the fear of failure and moving forward, you should ask yourself some honest questions:
- Do I care enough about this business concept to overcome the doubt and weather any storm, no matter what?
- Does my idea make me feel motivated and excited even when I think about the risks?
- Am I willing to keep learning, work late nights, and pick myself up after the setbacks?
Ask yourself these questions, and you'll land on an idea that starts as a small business but has the potential to scale while keeping you engaged and inspired in the long term.
Looking for inspiration? Check out this success story from entrepreneur and content creator, Lizzy Capri:
How Lizzy Capri Builds a Crazy Fun (& Successful) YouTube Channel | GoDaddy Makers
2. Use market research to validate your business potential
Something to consider when you’re starting your business is deciding how you will generate revenue. Doing that requires you to validate your idea.
So, if you're asking yourself, 'how do I start my own company?' and wondering what the next step is, the answer lies in market research.
To validate your concept, you must get under the skin of your industry and your target audience. In addition to gathering industry insights and data, you should build customer profiles and personas that represent your ideal target audience early in developing your business.
Here are the top three things you should consider when deciding whether there’s a market opportunity you can fill:
- Trends and timing: Is your business idea aligned with emerging business trends or current shifts in consumer behavior. Does your concept meet the needs of your audience and is there a need for it? Do your research and the answers will become clear.
- Competitor activity: A little competitor analysis will go a long way. Take the time to see what your potential competitors are offering, explore their marketing campaigns, and take to social media to see how they interact with their audience. Armed with this information, you’ll be able to see how you can offer something better and exploit any gaps in your competitors’ strategy.
- Pricing: Another key element to look for is how companies in your potential niche are pricing their products or services. Taking the time to do this will help you understand the value of what you’re offering and develop a sustainable pricing strategy.
For added wisdom, discover how vibrant culinary entrepreneur Linda Miller-Nicholson discover her niche and promoted it like a pro right here:
Finding Gold at the End of the Rainbow Pasta | GoDaddy Makers
3. Create a business plan
Once you've validated your business idea and you've got a handle on your target market, you'll need to start planning.
Your business plan is a valuable business resource that you can use to get external investment.
When starting a new business, developing your roadmap towards success is vital. Here are some tips to help you navigate the process like a boss:
- Consider your "why". This will help you gain an understanding of your key motivations for starting your business as well as what you want to achieve.
- Outline plans of action or initiatives that represent your brand mission and what you do.
- Define your key business goals using timelines and milestones to keep you on track.
- Write an executive summary that states all essential information related to your business's aims, goals, and products.
To streamline the process and get ahead of the competition, you can utilize AI tools like ChatGPT to gather feedback or ideas on your business plan. This popular generative AI platform can be a powerful tool for entrepreneurs looking to start a business, if you know how to use it with purpose.
Once you've gathered feedback and you're confident with your business plan's framework, you can use generative AI tools to help you write your business plan.
Whatever your business model, weaving AI into your marketing strategy will enhance your communications and boost your productivity. Go for it.
4. Get funding, explore financial options
To get your own business off to a flying start, you’re going to need to get the right level of funding. The funding options you explore will depend on the type of business you’re looking to start.
Let’s look at some of the funding options you can explore.
- Personal funds: If you’re wondering ‘how do I start a company and scale up?’, you can use your personal funds or savings to get up and running. Taking this route will give you total control on your decisions, empowering you to grow the business on your own terms. But, be aware: if your business struggles or fails to make a profit, you’ll be solely responsible for paying back any debt.
- Crowdfunding: You can use dedicated crowdfunding platforms to inspire people to donate to your business and offer a small stake or incentives in return. This can be an incredibly effective form of fundraising (especially as it builds excitement around your budding business)—and there have countless crowdfunding success stories over the years.
- Get a line of credit: A little like obtaining a personal line of credit, you can gain a line of business credit. The terms, limits, and interest rates will differ based on the nature of your business as well as factors like your credit rating or financial history.
- Gain a grant: Another solid way of securing a decent level of funding for your startup business is getting a grant. If your business mission is rooted in building a better community, doing good or inspiring innovation, you could be eligible for a grant from the Federal Government.
- Pitch to investors: Researching investors in your niche and pitching to them is a well-trodden way to raise funding in addition to professional backing, mentoring, and expertise. This is definitely an avenue worth exploring if you’re looking for startup capital, plus help with your business’s launch and development activities.
5. Choose a business structure
Before registering your business, settling on a water-tight legal structure is vital. Before you officially register your business, you have to decide on a concrete business structure — and the type you choose will impact your business from a legal standpoint. So, take your time when considering these structures.
Sole proprietorship
If you own your business independently, you can opt for sole proprietorship. This means that you will have full autonomy over your business, but you’ll be responsible for all debt, obligations, and finances.
A sole proprietorship is the easiest business legal structure to set up. If you have a little capital of your own, you can apply for a sole proprietorship with ease. You will have complete control over the entire business, but be aware: you will be responsible for the financial as well as legal aspects of the business.
Pros:
- You will be in full charge of business decisions, development and planning
- You will receive all of the business’s profits
- You will find filing for tax simpler
Cons:
- Managing everything on your own could burn you out and limit your potential for growth
- If you hit financial hot water, you will be liable for settling any debts or arrears
Partnership
Combining forces with another budding business owner will give you double the startup as well as another person who is liable for the red tape as well as the financial aspects of the business. More often than not, two heads are better than one — forge the right partnership and you could see your business thrive from the get-go.
Pros:
- You will have twice the skills, perspective, and financial scope
- You will have another person who is responsible for the running as well as financial and legal aspects of the business
Cons:
- If you and your partner disagree on any aspect, this could cause the kind of friction that could derail progress and stunt growth
Corporation
As a corporation, you will separate your personal assets from your business assets. This means that while your company can incur debt and be subject to legal disputes, in most cases your personal finances and assets will remain protected. There are many different forms of a corporation to consider, some of which offer access to some pretty decent investment opportunities.
Pros:
- You will gain access to excellent capital options
- You will benefit from certain tax breaks
- You will be able to protect your personal assets
Cons:
- Corporations can be costly to form and run
- There can be a lot of red tape involved in the running of a corporation
Limited liability company (LLC)
This type of business structure is one of the most common options for new businesses. As a limited liability company (LLC), you will benefit from the legal protection of a corporation while also reaping the tax rewards of a business partnership.
Pros:
- The setup process is relatively straightforward
- You will gain access to a healthy choice of capital and funding options
- You will have a certain level of protection concerning your personal finances
Cons:
- Your investment options can be limited with an LLC
- You can be subject to fairly costly annual maintenance fees
Want more information on how to start an LLC and the various types of LLC? Our guides have you covered.
Weigh up the pros and cons of each business structure, taking your aims and goals into consideration. At this point, you will want to brainstorm a catchy business name to make it official and bring your brand to life.Once you’ve decided on your brand-boosting business name, you secure the appropriate domain name.
6. Register your business and get the required licenses
The next step in how to start a business journey is registering your company and getting any required licenses.
By choosing your business name and settling on your business structure, you will have already started the registration process. To complete the registration process and obtain the right licenses, you will need to:
- Check the full registration requirements depending on your legal structure.
- Work through the registration requirements of any regions where you’re looking to trade or operate.
- Register for any taxes.
- Obtain an employee identification number (EIN), if necessary.
- File any relevant trademarks.
- Find out which business license is relevant to your organization and start the application process
Just remember, forming a business can be a bit complicated, so it's always a good idea to chat with a tax advisor or lawyer to make sure you're doing everything right.
Related: See How to Register a Business to access resource links to all 50 states.
7. Open a business bank account
Starting a business isn’t just about ideas and marketing—there are also certain administrative duties to consider—like opening a bank account.
The good news? In 2025, there is no end of choice when it comes to setting up a business bank account to keep your business and personal assets separate. Here are some of the additional benefits of opening a business bank account:
- Having a business banking account will make your outfit more professional and credible in the eyes of potential vendors or investors.
- You will find filing your taxes and carrying out accounting tasks cleaner, quicker, and easier.
- You may be eligible for bonuses like low (or no) monthly and higher transaction limits.
You should choose a bank account with benefits and features that suit your business’s size as well as your goals.
Tip: Create a shortlist of business bank accounts with flexible loan options as well as excellent customer service and online banking applications.
Checklist…
To set up your business bank account, you will need:
✔️ EaIN or tax ID numbers
✔️ Your official business name and location
✔️ Date the business was set up or established
✔️ Your Social Security number, address and date of birth
8. Get business insurance
Still pondering the question, “how do I open my own company correctly?” The next phase in the operation is getting insurance.
In addition to opening your bank account, you may also need to get business insurance. If you don’t, you could find yourself footing a colossal bill if any unexpected issues, damages or disputes arise.
Even when launching a small business, you will need to cover yourself with the right kind of insurance to protect yourself against any eventuality.
If you’re offering a service rather than tangible goods, it will also pay to get professional liability cover in case you make any consumer-facing mistakes.
Also, as your business scales, you might also consider employment practices liability insurance. This type of cover will protect you against any potential employee claim or complaint.
FYI: Business insurance costs will vary depending on your business size, set-up, and industry. According to Sofi, general and product liability insurance averages at an annual cost of $504 per year.
9. Establish your business's online identity
Next up in your start my own company quest, we come to networking and promotion. At this point, you will be ready to build your business’s online presence. Your website or online store will be the digital hub of your entire business—so getting the design and the functionality just right is essential.
If you’re interested in starting a website for your business, here are some hand-picked resources to help you out:
- How to start an online store
- How to do SEO: A beginner’s guide for small businesses and entrepreneurs
- 10 things every business owner should automate
- Guide to holiday marketing campaigns
Tip: To build an online store that is slick, wonderfully designed, and easy to navigate, try the powerful and easy-to-master GoDaddy Website Builder. Or build an online store with our slick and savvy ecommerce tool and tie your business together with a GoDaddy POS system to accept payments anywhere. GoDaddy also offers a suite of AI tools to help you take your idea online in minutes.
For inspiration on boosting your online presence and pushing for the professional burn, discover how Steven Davis grew Seattle’s Dave’s Fitness Method into the thriving business it is today:
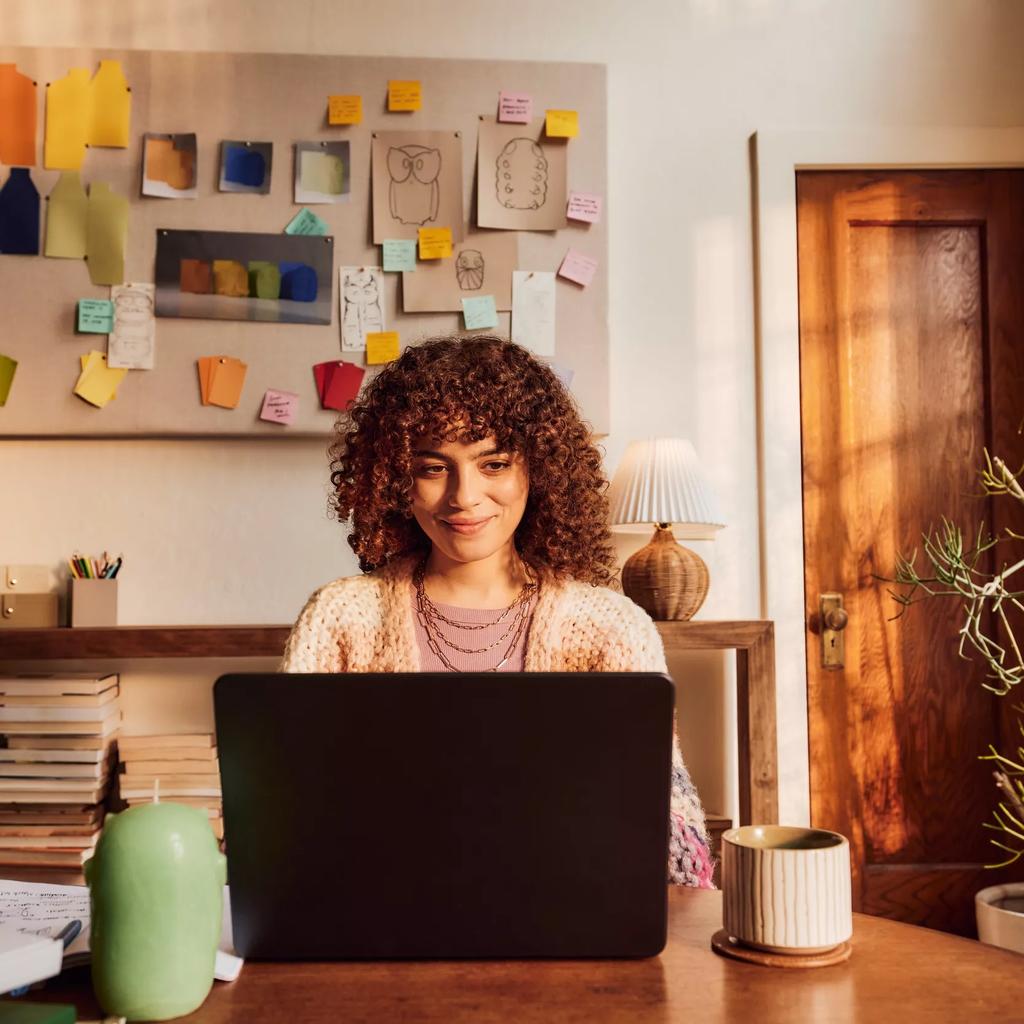
Go from idea to online in minutes with GoDaddy Airo™
Get started now.
10. Launch and grow your business
If you’ve followed all of the steps in this how to start a business in 2025 guide successfully, you’ll be ready to kick off.
Once you build a buzz about your big business launch across various channels including email and social media, you can start selling and take measures to grow year on year through marketing campaigns and sales initiatives.
To accelerate your commercial growth, there are measures you can take. These include:
Build relationships with early clients or customers
Those happy early customers will become your biggest brand advocates and will help you spread the word about your business.
To nurture those all-important early relationships you should:
- Send your existing customers personalized content, deals or recommendations
- Offer an exceptional customer experience (CX)
- Gather feedback to discover what’s working, what isn’t, and what needs improvement
- Get reviews and testimonials to build your trust and attract new customers to your business
Build strong partnerships
Collaborations can expand your brand’s reach and provide access to invaluable resources or expertise that can drive your business forward.
But what can you do to build solid partnerships? Let’s take a look:
- Look for (and connect with) complementary businesses and partner up for targeted co-promotions or bundled offers.
- Join local or industry-specific groups to connect with like-minded entrepreneurs and potential partners who can help you grow.
Get social
Don’t forget to create relevant social media profiles from the very beginning, and on other socials as well, if your audience uses them.
From LinkedIn to Instagram, getting active on social media will empower you to connect with potential clients or customers personally, share relevant content, and expand your brand reach. Here are some tips:
- Ask questions to encourage interaction, spark engagement, and spark up conversions with potential clients or customers.
- Create posts or share content that’s relevant to your audience’s needs and helps them solve their problems.
- Stay consistent, promote your latest news or offers, and make social media a core part of your business growth strategy.
To help you understand how to start a business on a deeper level, here are three additional resources to explore, fresh from the GoDaddy Resource Library:
- Take the 30 day ‘start a business’ challenge
- How to build a community as a business owner and why it matters
- How to implement a sustainable growth strategy — fast
Mistakes to avoid when you’re first starting out as a business owner
When starting a new business, knowing what not to do is as important as knowing what to do. That said, here are three common mistakes you should avoid.
Forgetting cash flow management
When you’re pondering the question, “how can I run a business sustainably”, understanding cash flow is important. If you don’t, you could run into financial hot water.
Your cash flow is the movement of money in and out of your business. By understanding the fundamental concepts of cash flow from the very start of your journey, you’ll keep your accounts accurate, your business buoyant, and your affairs in good shape.
So, take the time to understand the types of cash flow that applies to your business, put a plan in place, and keep your accounts up to date.
Ignoring customer feedback
Customer feedback is vital to your business as it gives you an opportunity to understand what you’re doing well and what needs improvement. A failure to gather customer feedback and put it into action could damage your brand reputation and have a negative impact on customer loyalty.
To gather customer feedback, you can set up a third-party review account (like Feefo or Trustpilot) to collect reviews and feedback. You can also incentivize your customers to take a survey to connect with detailed feedback on specific subjects like your new app, new products or your checkout experience.
Once you’ve gathered your feedback, categorize it and use these insights to take targeted measures that will improve what you offer your customers, build trust, and boost your bottom line.
Marketing consistently
Once they gain a little momentum, many small businesses start to slow down with their marketing efforts. That’s a big mistake.
Even when business is booming, you should take measures to promote your brand across channels. Keeping your marketing consistent will ensure that you can scale consistently.
Developing marketing campaigns and maintaining an online presence will also reduce the risk of you having dry spells or quiet periods.
Here are some measures you can take to keep your marketing initiatives flowing:
- Create seasonal marketing campaigns (Valentine’s Day, Easter, Christmas, etc.) to capture your audience’s attention and boost your sales.
- Consistently post relevant content across your most engaged social media channels.
- Set up an email list and deliver valuable content to your subscribers regularly to build trust and maintain engagement.
- Retarget your existing customers with tailored deals, incentives, and product recommendations
Starting a business in 2025 is a challenging but potentially rewarding venture. Be persistent, play to your strengths, take the time to ensure you’ve covered every base, and you’ll be winning on the commercial battlefield in no time. Best of luck.
FAQs
How can a beginner start a business?
A beginner can absolutely start a business. As long as you have a clear idea of your goals and a passion for what you do, you can make your business a real success. Follow the steps in this guide and you’ll be up and running sooner than you might think. Also, check out GoDaddy Airo, our integrated AI solution, and discover how it can help get your idea online in just minutes.
How much money do you need to start a business from scratch?
The cost of starting a business will vary depending on your industry or business model of choice. But, research from the US Small Business Administration shows that most small businesses cost around $3,000 to get started. And, the majority of home-based ventures cost $2,000 to $5,000 to set up. For larger ventures, there are several businesses that can be started with a budget of $10,000, such as a landscaping service or a small catering business. With careful planning and budgeting, entrepreneurs can successfully launch and grow their businesses without breaking the bank.
Can I run a business by myself?
There is no reason that you can’t run a small business by yourself. With the right resources and the right approach, you can set up, launch, and operate your business. Going solo is more than possible, but with so much to consider it’s a huge undertaking. As your business scales, hiring a small team or getting a partner on board will be a good idea. That way, you can further accelerate your commercial growth.
What is the easiest business to start?
A service-based business is often considered the easiest to start as you don’t have to deal in physical stock or inventory. But, in 2025, it’s never been more accessible to set up and launch a successful online business idea. In the digital age, tools exist to help you set up an ecommerce store or build a website without technical expertise. Follow the right steps and almost every kind of business will be within your reach.
How do I start my own business with no money?
Starting a business with no money may seem challenging, but it is possible with some creative thinking. Consider offering a service that requires minimal upfront costs, such as consulting or freelancing. Utilize free resources like social media and networking to promote your business. Look for potential investors or grants, and be willing to start small and grow gradually.
Can you start a side business while working full time?
Yes, it is possible to start a side business while working full time. Many people successfully manage their time and commitments by dedicating evenings and weekends to their side business. It may require careful planning and time management, but with dedication and perseverance, it is definitely achievable to juggle both a full-time job and a side business simultaneously.
Who can help me start a business?
There are many resources available to help you start a business. You can seek guidance from business advisors, mentors, or consultants who specialize in entrepreneurship. Local Small Business Development Centers (SBDCs) and Chambers of Commerce also offer valuable support and networking opportunities. Additionally, online platforms and communities provide access to a wealth of information and advice for aspiring entrepreneurs.
More Resources on Starting a Business
Here at the GoDaddy Garage, we pride ourselves on helping budding business owners get to where they deserve with an ever-growing library of tools and practical resources.
We help ambitious entrepreneurs by:
- Helping you decide whether you’re ready to be an entrepreneur
- Serving up practical video guides and success stories
- Launching innovative new products to supercharge your business success
- Telling stories and plain inspire you: